Creating a Dynamic Web Site using MySQL, PHP & Python Programming
Accredited by:
Android Authorized Training Centre (Android ATC)
Program Description:
PHP, MySQL & Python, are the most popular open source technologies to emerge during the past decade. PHP is a powerful language for writing server-sider Web applications. MySQL is the world’s most popular open source database. Together, these two technologies provide you with a powerful platform for building database-driven Web applications. This extraordinarily hands-on course teaches attendees, students, graduates everything they need to successfully build data-driven Web sites using PHP,MySQL & Python.
Module Outline:
LESSON 1 - Introduction
• The history and role of PHP
• The history and role of MySQL
• PHP architecture overview
• PHP basics
• Embedding PHP in HTML
• Using PHP tags
• PHP tag styles
• PHP statements
• Whitespace
• Comments
LESSON 2 - Adding dynamic content
• Accessing form variables
• Identifiers
• User-declared variables
• Assigning values to variables
• Variable types
• Constants
• Variable scope
• Operators
• Variable functions
• Control structures
• Conditionals
• Iteration and loops
• Breaking/continuing loops
LESSON 3 - Storing and retrieving data
• Saving data for later
• File processing overview
• Opening a file
• Writing to a file
• Closing a file
• Reading from a file
• Other useful file functions
• File locking
LESSON 4 - Working with Arrays
• Introduction to arrays
• Numerically indexed arrays
• Associative arrays
• Multidimensional arrays
• Sorting arrays
• Sorting multidimensional arrays
• Reordering arrays
• Loading arrays from files
• Other array manipulation
LESSON 5 - Working with String manipulation and regular expressions
• Formatting strings
• Joining and splitting strings via string functions
• Comparing strings
• Matching and replacing substrings with string functions
• Regular expressions: core syntax
• Finding and replacing substrings with regular expressions
• Extracting data from strings with regular expressions
LESSON 6 - Reusing code and writing functions
• Code reuse overview
• Using require() and include()
• Using require() for Web site templates
• Using functions in PHP
• Function structure, parameters, and variable scope
• Pass by reference versus pass by value
• Returning values from functions
• Recursion
LESSON 7 - Object-oriented PHP
• Object-oriented concepts
• Classes, attributes, and operations in PHP
• Instantiation
• Using class attributes
• Calling class operations
• Implementing inheritance
• Tips for proper class design
LESSON 8 - Building basic site functionality with PHP
• Processing forms
• Populating form elements
• Creating pages based on data in other files
LESSON 9 - Session control in PHP
• Overview of session control
• Session functionality and the role of cookies in sessions
• Implementing a simple session
• Configuring session control
• Using session control as an authentication mechanism
LESSON 10 - Introducing MySQL
• Overview of MySQL tools and capabilities
• Review of relational database concepts
• Database design tips
• Creating databases and users
• Users and privileges
• Setting up a user for the Web
• Creating tables
• MySQL identifiers
• Column data types
• Inserting, retrieving, updating, and deleting data in the database
LESSON 11 - Accessing MySQL from PHP
• Steps involved in querying a database from PHP
• Setting up the connection
• Choosing the database to use
• Querying the database
• Retrieving the results
• Disconnecting from the database
LESSON 12 - Inserting, updating, and deleting database records
• Review of SQL INSERT, UPDATE, and DELETE statements
• Building an effective record insertion interface
- Matching field lengths in the form to field lengths in the database
- Implementing client-side or serverside validation
- Inserting the record into the database
- Confirming the insertion
• Updating database records
- Creating the user interface for updating records
- Implementing client-side or serverside validation
- Updating the record
- Confirming the update
• Deleting database records
- Prompting the user to confirm the deleting
- Deleting the record
- Confirming the deletion of the record
LESSON 13 - Accessing stored procedures from PHP
• When to used stored procedures instead of ad hoc SQL queries
• Calling a stored procedure
• Passing input parameters to a stored procedure
• Receiving output parameters from a stored procedure
LESSON 14 - Managing date and time
• Getting the date and time from PHP
• Converting between PHP and MySQL date formats
• Date calculations
• Using the calendar functions
LESSON 15 - Generating email messages from PHP (e.g., for confi rmations)
• Script commands for sending email
• Configuring the to, cc, bcc, and subject lines of a message, as well as the body
• Attaching a file to a message
LESSON 16 - PHP debugging
• Programming errors
• Logic errors
Overview of DBA duties
-
Server startup/shutdown
-
Mastering the mysqladmin administrative client
-
Using the mysql interactive client
-
User account maintenance
-
Log file maintenance
-
Database backup/copying
-
Hardware tuning
-
Multiple server setups
-
Software updates and upgrades
-
File system security
-
Server security
-
Repair and maintenance
-
Crash recovery
-
Preventive maintenance
-
Understanding the mysqld server daemon
-
Performance analysis (isamchk & myisamchk)
Obtaining & Installing MySQL
-
Choosing what else to install (e.g. Apache, Perl +modules, PHP)
-
Which version of MySQL (stable, developer, source, binary)
-
Creating a user acccount for the mysql user and group
-
Download and unpack a distribution
-
Compile source code and install (or rpm)
-
Initialize the data directory and grant tables with mysql_install_db
-
Starting the server
-
Installing Perl DBI support
-
Installing PHP
-
Installing Apache
-
Obtaining and installing the samp_db sample database
The MySQL Data Directory
-
Deciding/finding the Data Directory's location
-
Structure of the Data Directory
-
How mysqld provides access to data
-
Running multiple servers on a single Data Directory
-
Database representation
-
Table representation (form, data and index files)
-
OS constraints on DB and table names
-
Data Directory structure and performance, resources, security
-
MySQL status files (.pid, .err, .log, etc)
-
Relocating Data Directory contents
Starting Up and Shutting Down the MySQL Server
-
Securing a new MySQL installlation
-
Running mysqld as an unprivileged user
-
Methods of starting the server
-
Invoking mysqld directly
-
Invoking safe_mysqld
-
Invoking mysql.server
-
Specifying startup options
-
Checking tables at startup
-
Shutting down the server
-
Regaining control of the server if you can't connect
Managing MySQL User Accounts
-
Creating new users and granting privileges
-
Determining who can connect from where
-
Who should have what privileges?
-
Administrator privileges
-
Revoking privileges
-
Removing users
Maintaining MySQL Log Files
-
The general log
-
The update log
-
Rotating logs
-
Backing up logs
Backing Up, Copying, and Recovering MySQL Databases
-
Methods: mysqldump vs. direct copying
-
Backup policies
-
Scheduled cycles
-
Update logging
-
Consistent and comprehensible file-naming
-
Backing up the backup files
-
Off-site / off-system backups
-
Backing up an entire database with mysqldump
-
Compressed backup files
-
Backing up individual tables
-
Using mysqldump to transfer databases to another server
-
mysqldump options (flush-logs, lock-tables, quick, opt)
-
Direct copying methods
-
Database replication (live and off-line copying)
-
Recovering an entire database
-
Recovering grant tables
-
Recovering from mysqldump vs. tar/cpio files
-
Using update logs to replay post-backup queries
-
Editing update logs to avoid replaying erroneous queries
Tuning the MySQL Server
-
Default parameters
-
The mysqladmin variables command
-
Setting variables (command line and options file)
-
Commonly used variables in performance tuning
-
back_log
-
delayed_queue_size
-
flush_time
-
key_buffer_size
-
max_allowed_packet
-
max_connections
-
table_cache
-
Erroneous use of record_buffer and sort_buffer
Running Multiple MySQL Servers
-
For test purposes
-
To overcome OS limits on per-process file descriptors
-
Separate servers for individual customers (e.g. ISPs)
-
Configuring and installing separate servers
-
Procedures for starting up multiple servers
Updating MySQL
-
Stable vs. development releases
-
Updates for both streams
-
Using the "Change Notes"
-
Bug fixing vs. new features
-
Dependencies on the MySQL C client library (PHP, Apache, Perl DBD::mysql)
MySQL Security
-
Assessing risks and threats
-
Internal security: data and directory access
-
Access to database files and log files
-
Securing both read and write access
-
Filesystem permissions
-
External security: network access
-
Structure and content of the MySQL Grant Tables
-
user, db, host, tables_priv, columns_priv
-
Grant table scope fields/columns
-
Grant table privilege columns
-
Database and table privileges: ALTER, CREATE, DELETE, DROP, INDEX, INSERT, SELECT, UPDATE
-
Administrative privileges: FILE, GRANT, PROCESS, RELOAD, SHUTDOWN
-
Server control over client access: matching grant table entries to client connection requests and queries
-
Scope column values: Host, User, Password, Db, Table_name, Column_name
-
Query access verification
-
Scope column mmatching order
-
Grant table risks: the FILE and ALTER privileges
-
Setting up users without GRANT
-
The anonymous user and sort order
MySQL Database Maintenance and Repair
-
Checking and repairing tables
-
Invoking myisamchk and isamchk
-
Extended checks
-
Standard table repair
-
Table repair with missing/damaged index or table description
-
Avoid server-checking interaction, without shutdowns
-
Internal and external locking
-
Locking for checks and locking for repairs
-
Speeding up checks
-
Scheduled checks and preventive maintenance with cron
-
Automated checks at startup
Introduction to Apache
1.1 History and Evolution
1.1.1 The Internet
1.1.2 The Hypertext Concept
1.1.3 The World Wide Web
1.2 The Apache Group
1.2.1 A Group of Volunteers
1.2.2 The Apache HTTP Server Project
1.2.3 The Apache Software Foundation
Apache Functionality
2.1 Apache Architecture
2.2 Apache Kernel Functionality
2.3 Apache Module Functionality
2.3.1 Core Functionality
2.3.2 URL Mapping
2.3.3 Access Control
2.3.4 User Authentication
2.3.5 Content Selection
2.3.6 Environment Creation
2.3.7 Server-Side Scripting
2.3.8 Response Header Generation
2.3.9 Internal Content Handlers
2.3.10 Request Logging
2.3.11 Experimental
2.3.12 Extensional Functionality
Building Apache
3.1 Sample Step-by-Step Installation
3.1.1 File System Preparation
3.1.2 Obtaining the Source Distribution
3.1.3 Package Prerequisites
3.1.4 Configuring Apache Source Tree
3.1.5 Building and Installing Apache
3.2 Configuration Reference
3.2.1 Configuration Variables
3.2.2 General Options
3.2.3 Stand-alone Options
3.2.4 Installation Layout Options
3.2.5 Build Options
3.2.6 suEXEC Options
3.3 Configuration Special Topics
3.3.1 Shadow Source Trees
3.3.2 On-the-Fly Addition of Third-Party Modules
3.3.3 Module Order and Permutations
Duration:
8 Days
Prerequisite:
-
Familiarity with the Windows operating system.
-
Familiarity with web terminology
-
Fluency in HTML and fundamentals of web coding
Training Method:
Instructor-Led-Hands-on-Practical Training , Workshop
Assessment Methods:
-
Pre-Test
-
Post-Test
-
Final Exam – Certification by MySQL & PP & I-World Technology TecTechnolohu
What is the core competency do I get by earning this certificate?
This extraordinarily hands-on course teaches attendees, students, graduates everything they need to successfully build data-driven Web sites using PHP and MySQL. Students, graduates, web professionals who need to develop dynamic web content through data driven pages, using PHP & MySQL. Experienced users will also benefit from the many tips and tricks, and special exercises.
What will I able to do as a result earning this certificate?
Student will able to;
How to set up PHP and MySQL on your server.
-
Programming fundamentals of the PHP language, including scalar variables, arrays, statements, conditionals, loops, and more.
-
Storing and retrieving data from files via PHP.
-
Advanced techniques for working with PHP arrays.
-
Techniques for using String functions and regular expressions to validate users’ form submissions and other data.
How will earning this certificate benefits me and my employer?
MySQL, PHP & Phyton language is required to create a dynamic website. By mastering it, participant will able to create advance and dynamic website to caters today clients. By mastering those languages, participant also can be a freelance webmaster.
Max Class Capacity:
20 - 30 persons.
Suitable For:
Public, Programming / Multimedia / IT related student, Web Programmer.
Career Pathway:
Website Developer, PHP & MySQL App Developer, PHP & MySQL App Dev Manager, PHP & MySQL Developer & Specialist.
Course Date:
To be adviced (TBA) - Please contact us.

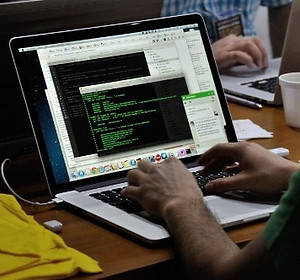